In an agile environment, features are implemented swiftly, and project scopes can change frequently. Developers write code that needs to meet criteria before code freezes – often leading to rushed work and resulting in inadequately reviewed solutions. The process of code review is critical to ensure meeting standards and quality. To enhance that process, it is best to automate as much of it as possible without eliminating the human element.
GitHub Actions offers a platform for teams to automate, customize, and execute software development workflows right from GitHub repositories. Teammates can automatically run tests, lint code, or even deploy applications. Automated control in deployments occurs when testing and linting act as gates. Actions is a powerful tool, offering great ways to enforce proper coding practices and conduct effective code reviews.
Here are a few ways to step up the code review game for projects:
- Enforce minimum coverage thresholds
- Avoids regression by exercising majority of the codebase
- Make meaningful changes to your codebase with an increased level of confidence
- Automate Pull Request (PR) reviewers from a random selection of experienced developers
- Emphasizes knowledge sharing of good coding standards
- Alleviates selection bias and the challenge of “who do I pick?”
- Shares accountability within the team
- Code Formatting and Linting Checks
- Verifies all code changes adhere to style guidelines
- Allows reviewers to focus on the logic and functionality of code
Enforcing Minimum Coverage Thresholds
Without a minimum coverage threshold, there may be functions, conditional branches, or edge cases that are not covered by tests. Regardless of if a code change is hastily made, the tests will help check that no bugs are inadvertently introduced, or any existing functionality is broken.
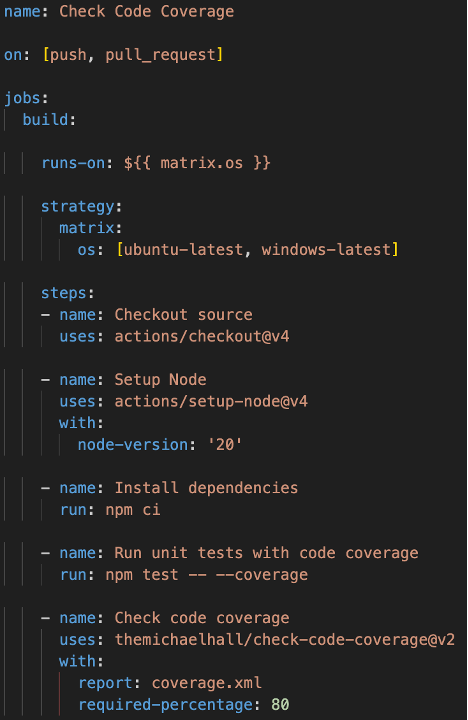
This example uses Node.js v20 and runs standard commands to prepare a repository for running tests on an Ubuntu and Windows machine for each commit, push, and pull request. Using an open-source action task on the Actions Marketplace, workflows will be marked as failed if the actual code coverage is below the specified 80%. If the tests are executed on another platform, this action can instead download the coverage report artifact and check against it. Other Node.js coverage commands like nyc or c8 can also gate coverage by percentages. Additionally, teams can confirm net new code is covered at or above thresholds using diff coverage.
Automating PR Reviewers
Let us say a team is refining its standards for a codebase and will benefit from a group of developers leading the refinement. Below is an example of a step that is executed when a PR is opened.
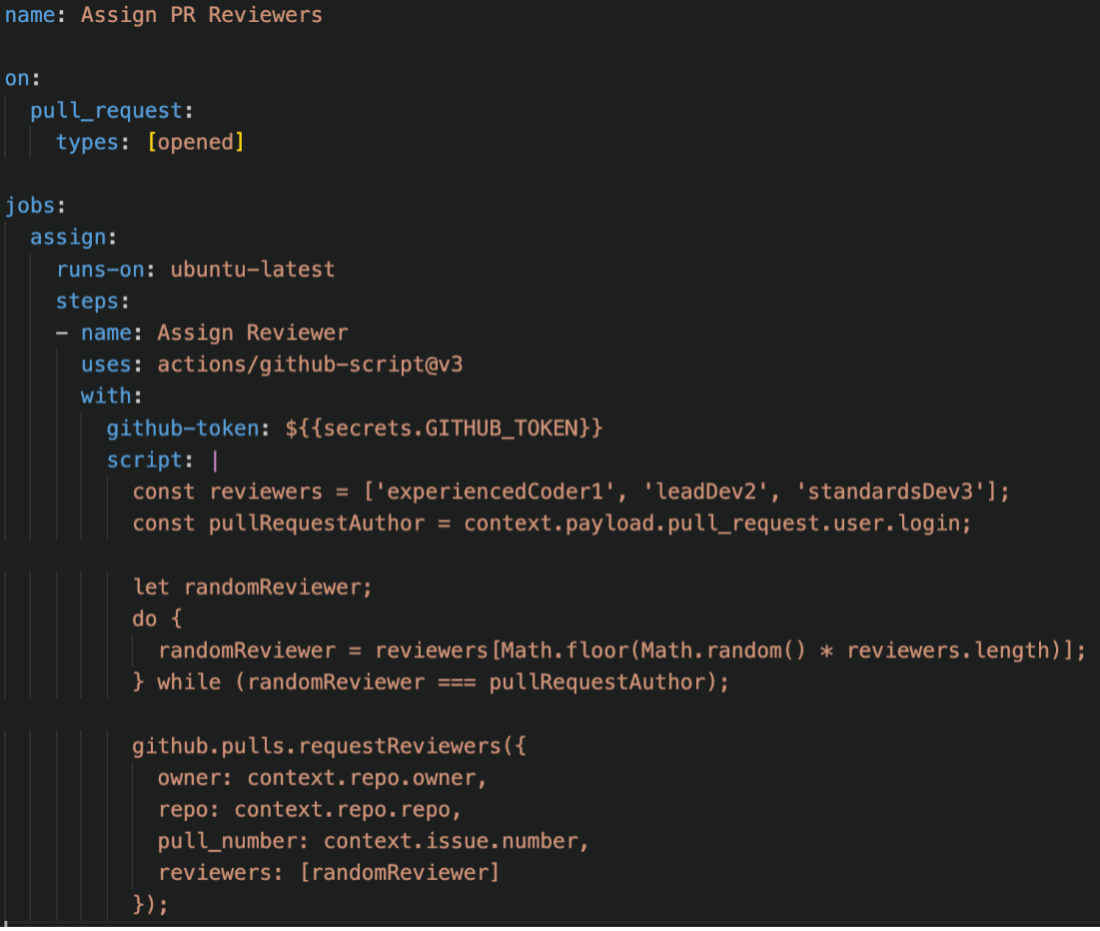
The lead developers still contribute to the codebase, so this step also will not try to add the author as the reviewer. This example has 3 fake GitHub IDs. For parts of the code that require specific business logic, pairing this GitHub Actions step with a CODEOWNERS file can amplify the confidence and acceptance of the changes in the PR.
Code Formatting and Linting Checks
Building off the previous tip around getting reviewers for your code, ensuring your code is readable and understandable is vital for an efficient review. IDEs offer excellent linting and styling baked in or with extensions. With GitHub Actions, you can make sure that all code adheres to a project’s style guidelines before code review begins. Automated linting checks allow reviewers to focus on the business logic, which is beneficial in inner-sourcing enterprise projects or in monorepos. A more succinct GitHub Actions code example is below.

This example uses a more shorthand flavor of declaring steps to run in a workflow. For a C# project, use .NET's built-in tools for formatting and linting. For other languages like Python, use packages like flake8 or black for the formatting.
Integrating these automated workflows into GitHub repositories will give teams technical advantage to bolster code review practices and uphold code standards.